1. Preparation
In this tutorial, I use OpenCV version 3.4.17 with Cuda v8.0 on Ubuntu 16.04, my GPU card is GT630 which is Fermi architecture. If you have newer card, you should re-compile your openCV to match with compute capability of your card and your Cuda version. Just change option -D CUDA_ARCH_BIN in your cmake command.
I will use Lenna image as test image for codes in this post.
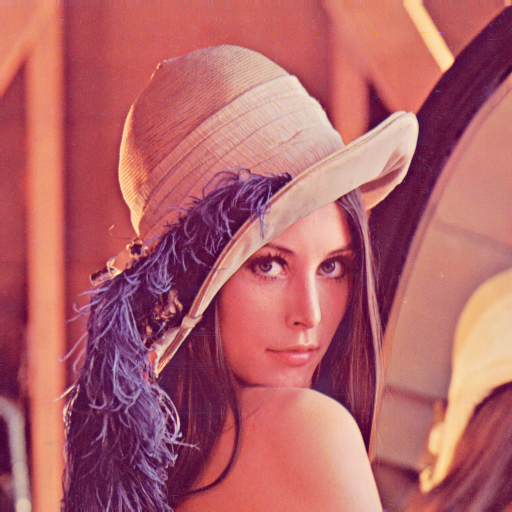
2. Adaptive Histogram Equalization (AHE)
Ordinary AHE tends to overamplify the contrast in near-constant regions of the image, since the histogram in such regions is highly concentrated. As a result, AHE may cause noise to be amplified in near-constant regions. Contrast Limited AHE (CLAHE) is a variant of adaptive histogram equalization in which the contrast amplification is limited, so as to reduce this problem of noise amplification (Wikipedia).
Create a new main
nano main.cpp
#include<opencv2/opencv.hpp>
using namespace cv;
using namespace cv::cuda;
int main(){
// Load image
Mat image = imread("lenna.png", IMREAD_GRAYSCALE);
imwrite("gray.png",image);
// Cuda mat
GpuMat dst, src;
// Contrast Limited Adaptive Histogram Equalization pointer
Ptr ptr_clahe = cuda::createCLAHE(5.0, Size(8, 8));
// Transfer image to CUDA
src.upload(image);
// Apply CLAHE
ptr_clahe->apply(src, dst);
// Get result back
Mat result;
dst.download(result);
// Write to file
imwrite("rs.png",result);
return 0;
}
Compile and test
ltkhanh@ServerTX:~/openCV$ g++ main.cpp $(pkg-config --libs --cflags opencv)
ltkhanh@ServerTX:~/openCV$ ./a.out
ltkhanh@ServerTX:~/openCV$
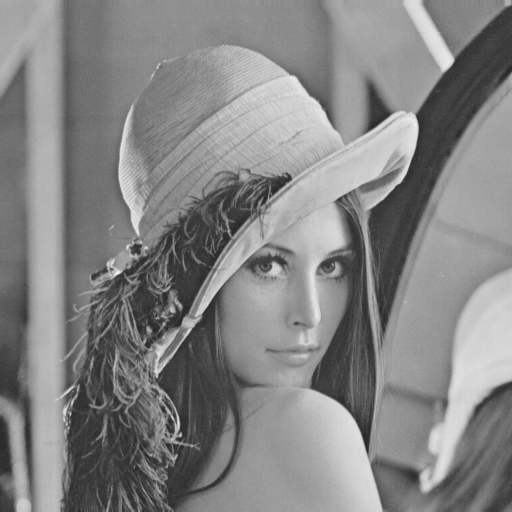
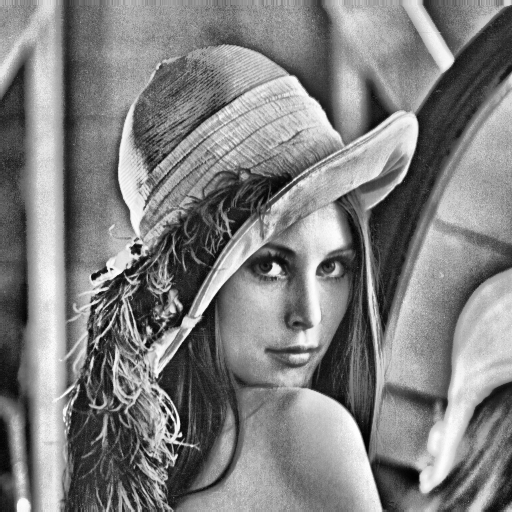
Leave a Reply